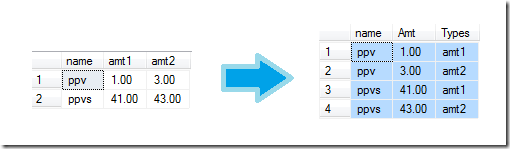
In a previous blog we converted rows into columns using pivot, now this time i needed to convert columns into rows. This was simply achieved using the unpivot functionality in sql server.
declare @tbl table(name varchar(50), amt1 numeric(12,2), amt2 numeric(12,2)) insert into @tbl(name, amt1, amt2)values('ppv', 1, 3) insert into @tbl(name, amt1, amt2)values('ppvs', 41, 43) select * from @tbl select * from @tbl unpivot ([Amt] for Types in (amt1, amt2)) as unpv
Credits:
Thanks to Sachin for this!!